HT16K33Segment14
This is a hardware driver for the SparkFun Qwiic Alphanumeric Display, which is based on the Freenove VK16K33, a clone of the Holtek HT16K33 controller. The driver communicates using I²C.
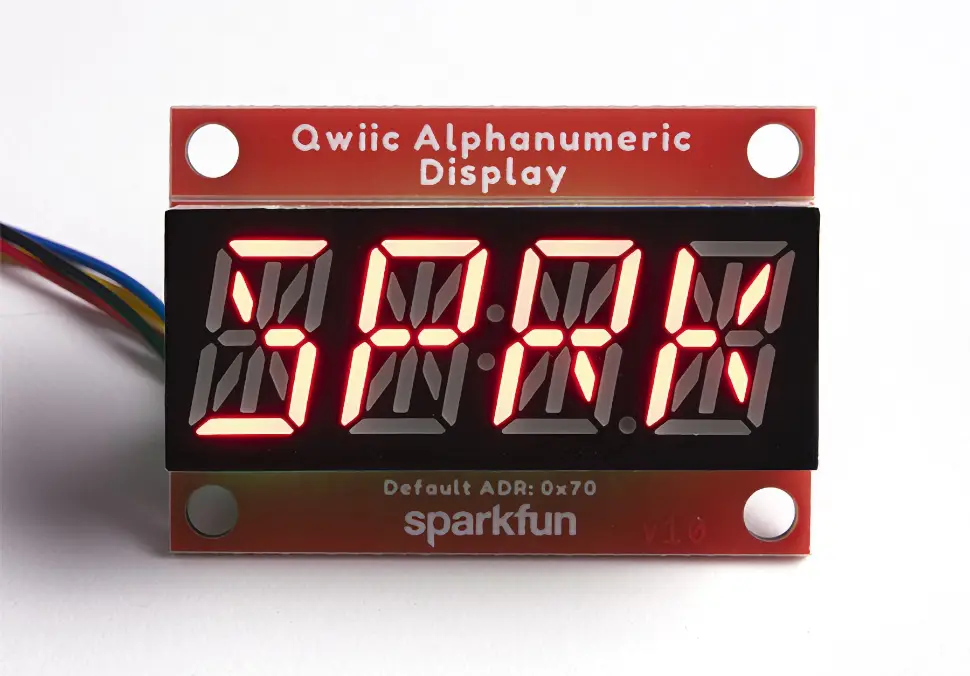
SparkFun Qwiic Alphanumeric Display. Image © SparkFun
It also supports the HT16K33-based Adafruit 0.54in Alphanumeric Display (version 3.2.0 and up) and the EC Buying 0.54in alphanumeric display.
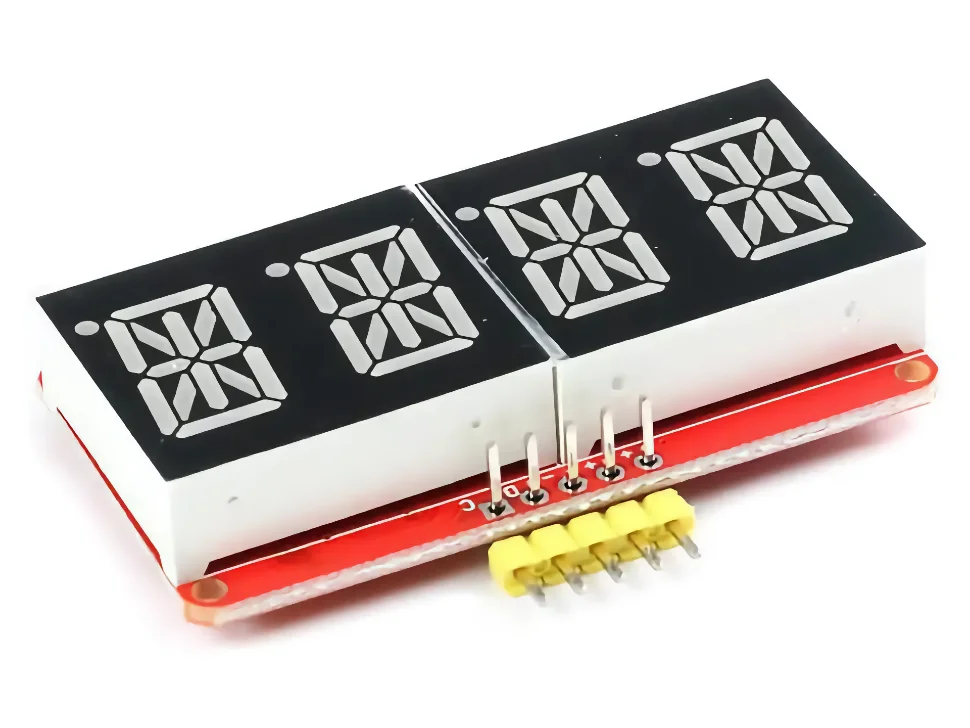
EC Buying Alphanumeric Display. Image © EC Buying
Compatibility
HT16K33Segment14
is compatible with MicroPython and CircuitPython.
Importing the Driver
The driver comprises a parent generic HT16K33 driver and a child driver for the 14-segment display itself. All your code needs to do is import the latter:
from ht16k33 import HT16K33Segment14
You can then instantiate the driver.
You will need both the display driver file and ht16k33.py in your project folder.
Characters
The class incorporates its own (limited) character set, accessed through the following codes:
Digits 0 through 9: codes 0 through 9
Characters A through Z: codes 10 through 35
Characters a through z: codes 36 through 61
Space character: code 62
Symbol characters: codes 63 through 75
| " ? $ % ° ' \ , * + - /
Display Digits
The display’s digits are numbered 0 to 3, from left to right.
Method Chaining
Most methods return a reference to the driver instance (self
) to allow method chaining with dot syntax:
led.clear().set_number(4, 0).set_number(3, 1).draw()
Class Constructor
- class HT16K33Segment14(i2C_bus, i2c_address=0x70, board=None)
To instantiate an HT16K33Segment14 object pass the I²C bus to which the display is connected and, optionally, its I²C address if you have changed the display’s address using the solder pads on rear of the LED’s circuit board.
The parameter
board
allows you to specify the type of display. Pass one of the following constants:Board
Constant
Adafruit
HT16K33Segment14.ADAFRUIT_054
SparkFun
HT16K33Segment14.SPARKFUN_ALPHA
EC Buying
HT16K33Segment14.ECBUYING_054
The passed I²C bus must be configured before the HT16K33Segment14 object is created.
- Parameters:
i2c_bus (I²C bus object) – The I²C bus to which the display is connected.
i2c_address (Integer) – An optional I²C address. Default:
0x70
.board (Int) – Constant indicating the type of board to be driven.
Examples
# MicroPython
from ht16k33 import HT16K33Segment14
from machine import I2C
# Update the pin values for your board
DEVICE_I2C_SCL_PIN = 5
DEVICE_I2C_SDA_PIN = 4
i2c = I2C(scl=Pin(DEVICE_I2C_SCL_PIN), sda=Pin(DEVICE_I2C_SDA_PIN))
led = HT16K33Segment14(i2c, board=HT16K33Segment14.SPARKFUN_ALPHA)
# CircuitPython - SparkFun Alphanumeric device
from ht16k33 import HT16K33Segment14
import busio
import board
i2c = busio.I2C(board.SCL, board.SDA)
while not i2c.try_lock():
pass
led = HT16K33Segment14(i2c, board=HT16K33Segment14.SPARKFUN_ALPHA)
# Circuitpython - Adafruit 0.54in device
from ht16k33 import HT16K33Segment14
import busio
import board
i2c = busio.I2C(board.SCL, board.SDA)
while not i2c.try_lock():
pass
led = HT16K33Segment14(i2c, board=HT16K33Segment14.ADAFRUIT_054)
Class Methods
- HT16K33Segment14.set_brightness(brightness=15)
Set the LED’s brightness (its duty cycle). If you don’t pass a value, the method will default to maximum brightness.
- Parameters:
brightness (Integer) – An optional brightness value between 0 (dim) and 15 (maximum brightness). Default: 15.
Example
# Turn down the display brightness
led.set_brightness(1)
- HT16K33Segment14.set_blink_rate(rate=0)
This method can be used to flash the display.
The value passed into
rate
is the flash rate in Hertz. This value must be one of the following values, fixed by the HT16K33 controller: 0.5Hz, 1Hz or 2Hz. You can also pass in 0 to disable flashing, and this is the default value.- Parameters:
rate (Integer/Float) – The flash rate in Hertz. Default: 0.
Example
# Blink the display every second
led.set_blink_rate(1)
- HT16K33Segment14.set_colon(is_set=True)
Specify whether the display’s center colon symbol is illuminated (
True
) or not (False
).This method is available only for VK16K33-based displays, in particular the SparkFun Qwiic Alphanumeric Display, and just returns
self
for other controller types.- Parameters:
is_set (Bool) – Is the colon set? Default:
True
.- Returns:
The instance (
self
).
Example
# Set the display to --:--
led.set_character("-", 0).set_character("-", 1).set_character("-", 2).set_character("-", 3)
led.set_colon().draw()
- HT16K33Segment14.set_decimal(is_set=True)
Specify whether the display’s decimal point, between digits 2 and 3,is illuminated (
True
) or not (False
).This method is available only for VK16K33-based displays, in particular the SparkFun Qwiic Alphanumeric Display, and just returns
self
for other controller types.- Parameters:
is_set (Bool) – Is the decimal point set? Default:
True
.- Returns:
The instance (
self
).
Example
# Set the display to 042.0
led.set_character("0", 0).set_character("4", 1).set_character("2", 2).set_character("0", 3)
led.set_colon().draw()
- HT16K33Segment14.set_glyph(glyph, digit=0, has_dot=False)
Write a character that is not in the character set — see Characters, above — to a specified digit.
Calculate the glyph pattern value using the following chart. The segment number is the bit that must be set to illuminate it (or unset to keep it unlit):
0 9 - 5 | | 1 8 \ | / 10 | | \|/ 6 - - 7 4 | | 2 /|\ | _ | 13 / | \ 11 . 14 3 12
Bit 14 is the period, but this is set with parameter 3 (HT16K33 only; for the VK16K33, see set_decimal).
Nb. Bit 15 is not read by the display.
For example, to define the letter
P
, we need to set segments 0, 4, 5, 6 and 8. In bit form that makes0x171
, and this is the value passed intoglyph
.Important This is the encoding for the VK16K33. For the HT16K33, bits 11 and 13 are swapped. For pre-defined characters, the driver swaps these bits this for you.
- Parameters:
glyph (Integer) – A glyph-definition pattern.
digit (Integer) – The digit to show the number. Default: 0.
has_dot (Bool) – Should the adjacent decimal point be set? Default: False
- Returns:
The instance (
self
).
- HT16K33Segment14.set_character(character, digit=0, has_dot=False)
Write a character from the display’s hexadecimal character set to a single digit, call set_character() and pass the the letter to be displayed (
"0"
to"9"
,"A"
to"Z"
,"a"
to"z"
) and the digit number (0, 1, 2 or 3, left to right) as its parameters. You can pass the string"deg"
for a degree symbol.If you need other letters or symbols, these can be generated using set_glyph().
- Parameters:
character (String) – The alphanumeric character to display.
digit (Integer) – The digit to show the number. Default: 0.
has_dot (Bool) – Should the adjacent decimal point be set? Default: False
- Returns:
The instance (
self
).
Example
# Display 'bEEF' on the LED
led.set_character("b", 0).set_character("e", 1)
led.set_character("e", 2).set_character("f", 3).draw()
- HT16K33Segment.set_number(number, digit=0, hasDot=False)
Write a single-digit number from the display’s hexadecimal character set to a single display digit.
Pass the the value you need as 0-15 or
0x00
-0x0F`
. If you need other letters or symbols, these can be generated using set_glyph().By default, the decimal point is not lit. For KT16H33 displays, see set_decimal.
- Parameters:
character (String) – The number to display.
digit (Integer) – The digit to show the number. Default: 0.
has_dot (Bool) – Is the character’s decimal point set? Default:
False
.
- Returns:
The instance (
self
).
Example
# Display '0042' on the LED
led.set_number(0, 0).set_number(0, 1)
led.set_number(4, 2).set_number(2, 3).draw()
- HT16K33Segment14.clear()
Wipe the class’ internal display buffer.
clear()
does not update the display, only the buffer. Call draw() to refresh the LED.- Returns:
The instance (
self
).
Example
# Clear the display
led.clear().draw()
- HT16K33Segment14.draw()
Call draw()
after changing any or all of the internal display buffer contents in order to reflect those changes on the display itself.