HT16K33MatrixMulti
This is a hardware driver for multiple 8x8 matrix LEDs (such as the Adafruit 1.2-inch 8x8 monochrome LED matrix backpack) all based on the Holtek HT16K33 controller and mounted alongside each other to form a composite display. The driver communicates using I²C.
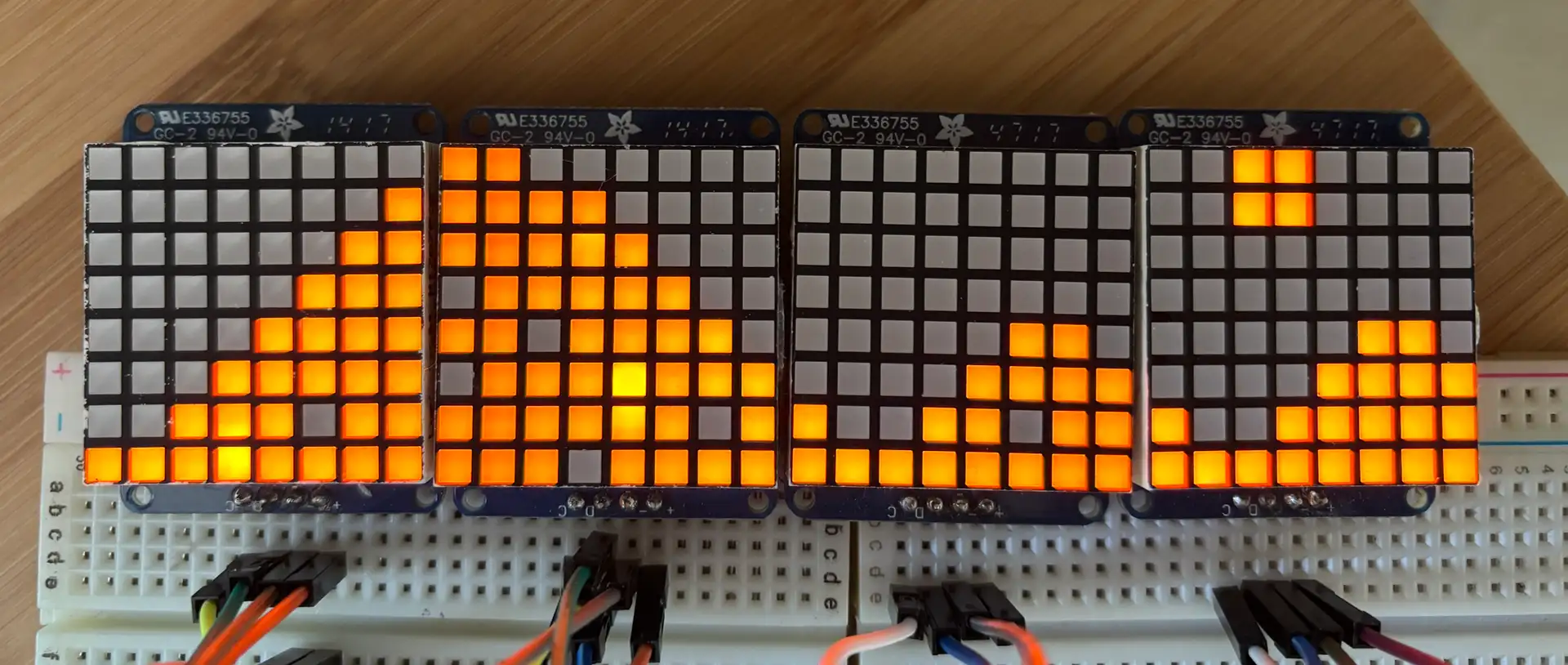
A typical multi-matrix display. Image © Tony Smith
Compatibility
HT16K33MatrixMulti
is compatible with MicroPython and CircuitPython.
Importing the Driver
from ht16k33 import HT16K33MatrixMulti
You can then instantiate the driver.
You will need both the display driver file (ht16k33matrixmulti.py
), the generic HT16K33 driver (ht16k33.py
) and the HT16K33Matrix driver (ht16k33matrix.py
) in your project folder.
Class Constructor
- class HT16K33MatrixMulti(i2C_bus, count, addresses=[])
To instantiate an HT16K33MatrixMulti object pass the I²C bus to which the display is connected and the number of matrix LEDs in your composite display.
The driver assumes the LEDs are mounted left to right, with the addresses
0x70
and up in sequence. If your LEDs do not use this sequence, use theaddresses
parameter and pass in an array of I²C addresses. The order of addresses must match the order of LEDs they refer to.The passed I²C bus must be configured before the HT16K33MatrixMulti object is created.
- Parameters:
i2c_bus (I²C bus object) – The I²C bus to which the display is connected.
count (Integer) – The number of matrix LEDs in your display.
addresses (Array of integers) – An optional array of I²C addresses.
Examples
# MicroPython
from ht16k33 import HT16K33MatrixMulti
from machine import I2C
# Update the pin values for your board
DEVICE_I2C_SCL_PIN = 5
DEVICE_I2C_SDA_PIN = 4
i2c = I2C(scl=Pin(DEVICE_I2C_SCL_PIN), sda=Pin(DEVICE_I2C_SDA_PIN))
display = HT16K33MatrixMulti(i2c, 4)
# CircuitPython
from ht16k33 import HT16K33MatrixMulti
import busio
import board
i2c = busio.I2C(board.SCL, board.SDA)
while not i2c.try_lock():
pass
display = HT16K33MatrixMulti(i2c, 4)
Class Methods
- HT16K33MatrixMulti.set_brightness(brightness=15)
Set the display’s brightness (its duty cycle). If you don’t pass a value, the method will default to maximum brightness.
- Parameters:
brightness (Integer) – An optional brightness value between 0 (dim) and 15 (maximum brightness). Default: 15.
Example
# Turn down the display brightness
display.set_brightness(1)
- HT16K33MatrixMulti.scroll_text(the_line, speed=0.1, do_loop=False)
Write the supplied ext to the display and scroll it right to left.
Optionally, you can also set the speed of the scroll by providing the period in seconds for which the code will pause between animation frames.
You can also specify whether you want to animation to auto-repeat after it has completed.
- Parameters:
the_line (String) – The text message to scroll across the display.
speed – The inter-frame pause period. The lower the value, the faster the scroll. Default: 0.1 seconds.
do_loop – Should the animation auto-repeat. Default:
False
.
Example
# Display the result
display.scroll_text("Well done! You scored: {}".format(game_score))
- HT16K33MatrixMulti.scroll_image(the_image, speed=0.1, do_loop=False)
Write the supplied image data to the display and scroll it right to left.
Optionally, you can also set the speed of the scroll by providing the period in seconds for which the code will pause between animation frames.
You can also specify whether you want to animation to auto-repeat after it has completed.
- Parameters:
text – The image to scroll across the display.
speed – The inter-frame pause period. The lower the value, the faster the scroll. Default: 0.1 seconds.
do_loop – Should the animation auto-repeat. Default:
False
.
Example
# Display the landscape
landscape = [0x01,0x03,0x07,0x0F,0x1D,0x3F,0x7F,0xEB,0xFF,0x77,0x3E,0x1F,0x0D,0x07,0x03,0x01,
0x01,0x03,0x07,0x0D,0x0F,0x07,0x03,0x01,0x01,0x03,0x07,0x0F,0x1D,0x3F,0x7F,0xEB,
0xFF,0x77,0x3E,0x1F,0x0D,0x07,0x03,0x01,0x01,0x03,0x07,0x0D,0x0F,0x07,0x03,0x01,
0xC1,0xC3,0x07,0x0F,0x0F,0x07,0x03,0x01]
landscape_bytes = bytes(landscape)
display.scroll_image(landscape_bytes)
- HT16K33MatrixMulti.clear()
Wipe the class’ internal display buffer.
clear()
does not update the display, only the buffer.
Example
# Clear the display
display.clear()