HT16K33Bar
This is a hardware driver for the Bi-Color (Red/Green) 24-Bar Bargraph w/I2C Backpack, which is based on the Holtek HT16K33 controller. The driver communicates using I²C.
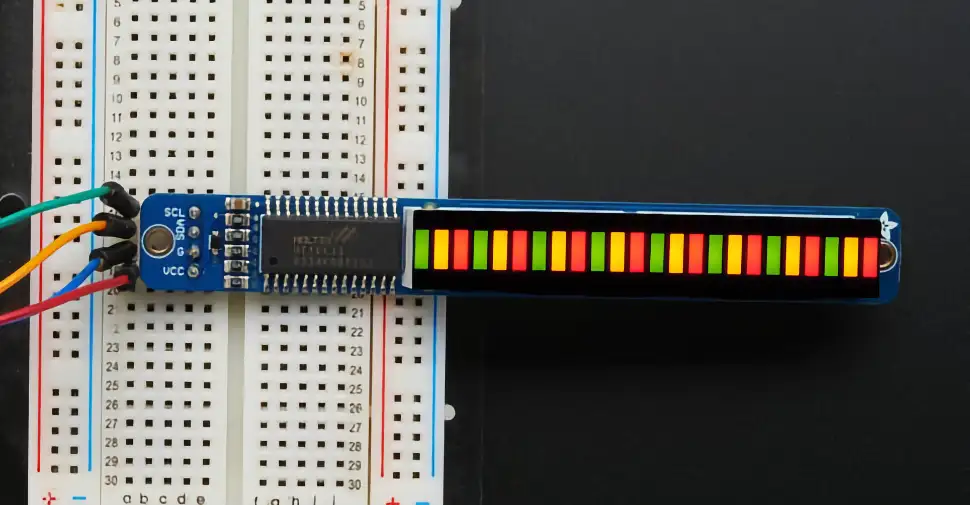
Adafruit Bi-Color (Red/Green) 24-Bar Bargraph w/I2C Backpack. Image © Adafruit
Attention
This functionality requires version 4.1.0 or above of the HT16K33 library.
Compatibility
HT16K33Bar
is compatible with MicroPython and CircuitPython.
Importing the Driver
The driver comprises a parent generic HT16K33 driver and a child driver for the bar graph display itself. All your code needs to do is import
the latter:
from ht16k33 import HT16K33Bar
You can then instantiate the driver.
You will need both the ht16k33bar.py
driver file and ht16k33.py
in your project folder.
Orientation
The Adafruit bar graph can be oriented in one of two ways: with the lowest bar (0) adjacent to the HT16K33 chip and the highest (23) at the far end of the display, or with the highest bar adjacent to the HT16K33. Specify which way up your bar graph is oriented with the constructor’s orientation
parameter. This takes one of two possible values: BAR_ZERO_ALONGSIDE_CHIP
and BAR_ZERO_FURTHEST_FROM_CHIP
. The former is the default.
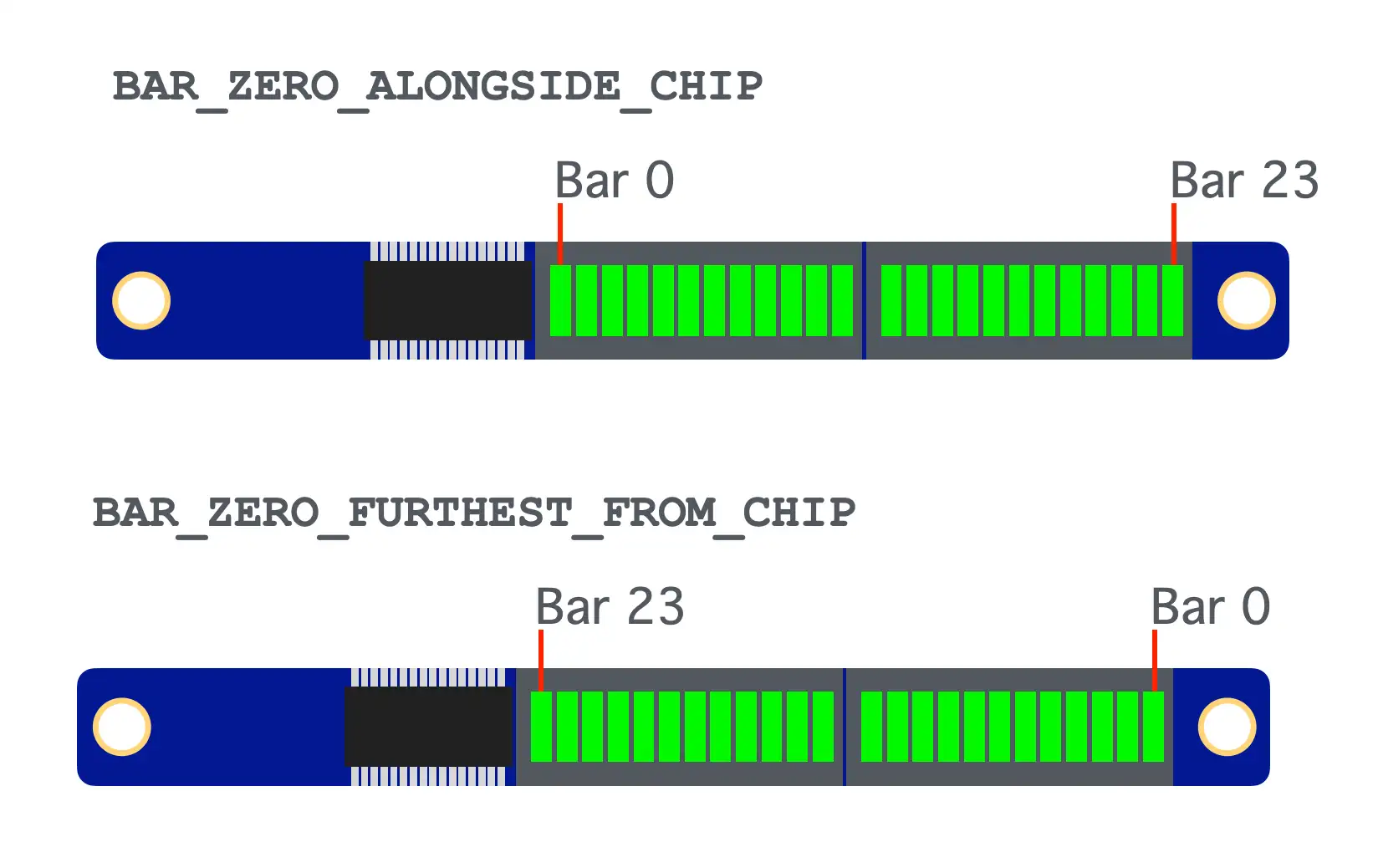
Note
If you are using a self-designed bar graph, or one from a different vendor, you may need to experiment to determine the native orientation of the display.
Colours
The bi-colour LED elements are capable of showing three colours (and unlit): green, red and yellow. Specify these colours using the following constants that are properties of the HT16K33Bar instance:
BAR_COLOUR_OFF
/BAR_COLOUR_CLEAR
BAR_COLOUR_GREEN
BAR_COLOUR_RED
BAR_COLOUR_YELLOW
/BAR_COLOUR_AMBER
Method Chaining
Most methods return a reference to the driver instance (self
) to allow method chaining with dot syntax:
graph.clear().plot(0,1,0).draw()
Class Constructor
- class HT16K33Bar(i2C_bus, i2c_address=0x70, orientation=BAR_ZERO_ALONGSIDE_CHIP)
To instantiate an HT16K33Bar object pass the I²C bus to which the display is connected and, optionally, its I²C address if you have changed the display’s address using the solder pads on rear of the LED’s circuit board.
The passed I²C bus must be configured before the HT16K33Bar object is created.
- Parameters:
i2c_bus (I²C bus object) – The I²C bus to which the display is connected.
i2c_address (Integer) – An optional I²C address. Default:
0x70
.orientation (Integer) – An optional orientation constant. Default:
BAR_ZERO_ALONGSIDE_CHIP
.
Examples
# MicroPython
from ht16k33 import HT16K33Bar
from machine import I2C
Update the pin values for your board
DEVICE_I2C_SCL_PIN = 5
DEVICE_I2C_SDA_PIN = 4
i2c = I2C(scl=Pin(DEVICE_I2C_SCL_PIN), sda=Pin(DEVICE_I2C_SDA_PIN))
graph = HT16K33Bar(i2c)
# CircuitPython
from ht16k33 import HT16K33Bar
import busio
import board
i2c = busio.I2C(board.SCL, board.SDA)
while not i2c.try_lock():
pass
graph = HT16K33Bar(i2c)
Class Methods
- HT16K33Bar.set_brightness(brightness=15)
Set the LED’s brightness (its duty cycle). If you don’t pass a value, the method will default to maximum brightness.
- Parameters:
brightness (Integer) – An optional brightness value between 0 (dim) and 15 (maximum brightness). Default: 15.
Example
# Turn down the display brightness
graph.set_brightness(1)
- HT16K33Bar.set_blink_rate(rate=0)
This method can be used to flash the display.
The value passed into
rate
is the flash rate in Hertz. This value must be one of the following values, fixed by the HT16K33 controller: 0.5Hz, 1Hz or 2Hz. You can also pass in 0 to disable flashing, and this is the default value.- Parameters:
rate (Integer/Float) – The flash rate in Hertz. Default: 0.
Example
# Blink the display every second
graph.set_blink_rate(1)
- HT16K33Bar.set(bar, colour)
Set a specific bar (in the range 0-23 for a single backpack) to the specified colour. Set the colour to
HT16K33Bar.BAR_COLOUR_CLEAR
to turn the bar off.set()
does not update the display, only the buffer. Call draw() to refresh the LED.- Parameters:
bar (Integer) – The index of the bar along the graph.
colour (Integer) – The chosen colour.
- Returns:
The instance (
self
).
Example
# Display a green bar half way along
graph.set(12, graph.BAR_COLOUR_GREEN).draw()
- HT16K33Bar.fill(bar, colour)
Fill the graph up to and including the specified bar (in the range 0-23 for a single backpack) with the specified colour. Set the colour to
HT16K33Bar.BAR_COLOUR_CLEAR
to turn the area off.fill()
does not update the display, only the buffer. Call draw() to refresh the LED.- Parameters:
bar (Integer) – The index of the bar along the graph.
colour (Integer) – The chosen colour.
- Returns:
The instance (
self
).
Example
# Fill the bottom half of the graph
graph.fill(12, graph.BAR_COLOUR_YELLOW).draw()
- HT16K33Bar.clear()
Wipe the class’ internal display buffer.
clear()
does not update the display, only the buffer. Call draw() to refresh the LED.- Returns:
The instance (
self
).
Example
# Clear the display
graph.clear().draw()
- HT16K33Bar.draw()
Call
draw()
after changing any or all of the internal display buffer contents in order to reflect those changes on the display itself.